文章目录
- 前言
-
- 一、安装turf
- 二、加载高德
- 三、绘制图形
- 四、计算交点
- 五、编写获取子多边形的函数
- 六、调用分割函数并绘制图像
- 七、效果
前言
分割矢量多边形
一、安装turf
npm i @turf/turf
二、加载高德
AMapLoader.load({
key: "你的key", // 申请好的Web端开发者Key,首次调用 load 时必填
version: "2.0", // 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
plugins: ["AMap.PolygonEditor", "AMap.LngLat", "AMap.Polygon"], // 需要使用的的插件列表,如比例尺'AMap.Scale'等
}).then((AMap) => {
//此处处理后续操作
}
三、绘制图形
map = new AMap.Map("container", {
// 设置地图容器id
viewMode: "3D", // 是否为3D地图模式
zoom: 13, // 初始化地图级别
center: [116.471354, 39.994257],
});
// 绘制折线
var polylinePath = [
[116.478, 40.000296],
[116.478603, 39.997534],
[116.478, 40.000296],
];
// 创建折线实例
var polyline = new AMap.Polyline({
path: polylinePath,
strokeColor: "#FF0000",
strokeWeight: 5,
lineCap: "round",
});
map.add(polyline); // 添加至地图
// 绘制多边形
var polygonPath = [
[116.475334, 39.997534],
[116.476627, 39.998315],
[116.478603, 39.99879],
[116.478529, 40.000296],
[116.475082, 40.000151],
[116.473421, 39.998717],
[116.475334, 39.997534],
];
// 创建多边形实例
var polygon = new AMap.Polygon({
path: polygonPath,
fillColor: "#FFC0CB",
strokeColor: "#000000",
strokeWeight: 2,
});
map.add(polygon); // 添加至地图
四、计算交点
// 格式转化
// 转换折线为Turf线段
var turfPolyline = turf.lineString(polylinePath.map((p) => [p[0], p[1]]));
// 转换多边形为Turf多边形
var turfPolygon = turf.polygon([polygonPath.map((p) => [p[0], p[1]])]);
// // 计算折线与多边形的交点
var intersections = turf.lineIntersect(turfPolyline, turfPolygon);
// 获取交点数量
var intersectionCount = intersections.features.length;
console.log("交点个数:", intersectionCount);
console.log(
"交点:",
intersections.features.map((i) => i.geometry.coordinates)
);
五、编写获取子多边形的函数
function polygonCut(
poly,
line,
tolerance = 0.001,
toleranceType = "kilometers"
) {
// 1. 条件判断
if (poly.geometry === void 0 || poly.geometry.type !== "Polygon")
throw "传入的必须为polygon";
if (
line.geometry === void 0 ||
line.geometry.type.toLowerCase().indexOf("linestring") === -1
)
throw "传入的必须为linestring";
if (line.geometry.type === "LineString") {
if (
turf.booleanPointInPolygon(
turf.point(line.geometry.coordinates[0]),
poly
) ||
turf.booleanPointInPolygon(
turf.point(
line.geometry.coordinates[line.geometry.coordinates.length - 1]
),
poly
)
)
throw "起点和终点必须在多边形之外";
}
// 2. 计算交点,并把线的点合并
let lineIntersect = turf.lineIntersect(line, poly);
const lineExp = turf.explode(line);
for (let i = 0; i < lineExp.features.length - 1; i++) {
lineIntersect.features.push(
turf.point(lineExp.features[i].geometry.coordinates)
);
}
// 3. 计算线的缓冲区
const lineBuffer = turf.buffer(line, tolerance, {
units: toleranceType,
});
// 4. 计算线缓冲和多边形的difference,返回"MultiPolygon",所以将其拆开
var _body = turf.difference(turf.featureCollection([poly, lineBuffer]));
console.log(_body, 134);
let pieces = [];
if (_body.geometry.type === "Polygon") {
pieces.push(turf.polygon(_body.geometry.coordinates));
} else {
_body.geometry.coordinates.forEach(function (a) {
pieces.push(turf.polygon(a));
});
}
return pieces;
}
六、调用分割函数并绘制图像
let polygons = polygonCut(turfPolygon, turfPolyline);
// 绘制子多边形(根据需求将数据保存只后台)
var polygon1 = new AMap.Polygon({
path: [polygons[0].geometry.coordinates],
fillColor: "red",
strokeColor: "#000000",
strokeWeight: 2,
});
map.add(polygon1); // 添加至地图
var polygon2 = new AMap.Polygon({
path: [polygons[1].geometry.coordinates],
fillColor: "green",
strokeColor: "#000000",
strokeWeight: 2,
});
map.add(polygon2); // 添加至地图
七、效果
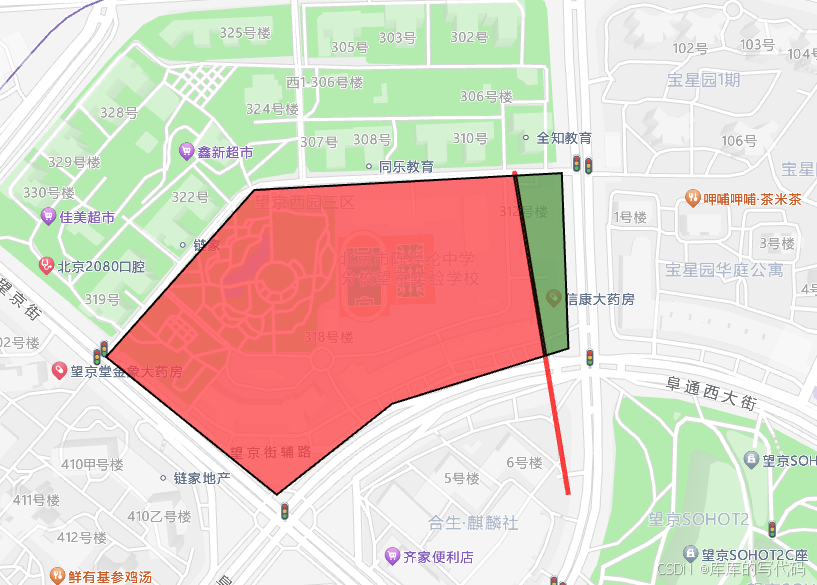