LeetCode热题100精讲——Top7:接雨水【双指针】
|
文章目录
- 题目背景
- 接雨水
- C++解法
- Python解法
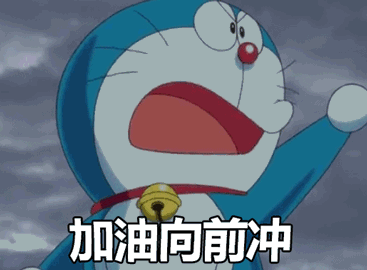
题目背景
如果大家对于 双指针 的概念并不熟悉, 可以先看我之前为此专门写的算法详解:
蓝桥杯算法竞赛系列第七章——六道力扣经典带你刷爆双指针
这题和前面做的LeetCode热题100精讲——Top5:盛最多水的容器【双指针】很相似, 感兴趣的老铁可以先把这一题做了再来看下面这一题哦.
接雨水
题目链接: 接雨水
解题思路: 参考学习《la bu la dong》
本题主要难点是对于任意位置 i , 能够装的水:
water[i] = min( # 左边最高的柱子 max(height[0..i]), # 右边最高的柱子 max(height[i..end]) ) - height[i]
关键在于, 如何能够计算出某一个位置左侧所有柱子的最大高度和右侧所有柱子的最大高度.
C++解法
class Solution {
public:
int trap(vector<int>& height)
{
// 定义双指针
int left = 0, right = height.size() - 1;
int res = 0;
// 记录左右两侧最高柱子的高度
int lmax = 0, rmax = 0;
while(left < right)
{
// 更新左右两侧最高柱子的高度
lmax = max(lmax, height[left]);
rmax = max(rmax, height[right]);
// 移动双指针 - 低的一端移动
// res = min(lmax, rmax) - height[i]
if(lmax < rmax)
{
res += lmax - height[left];
left++;
}
else
{
res += rmax - height[right];
right--;
}
}
return res;
}
};
Python解法
class Solution:
def trap(self, height: List[int]) -> int:
# 定义双指针
left, right = 0, len(height) - 1
lmax, rmax = 0, 0 # 左右两侧最高柱子的高度
res = 0 # 记录结果
while left < right:
lmax = max(lmax, height[left])
rmax = max(rmax, height[right])
# 移动双指针 - 低的一端移动
# res = min(lmax, rmax) - height[i]
if height[left] < height[right]:
res += lmax - height[left]
left += 1
else:
res += rmax - height[right]
right -= 1
return res
|
|