一.连接点
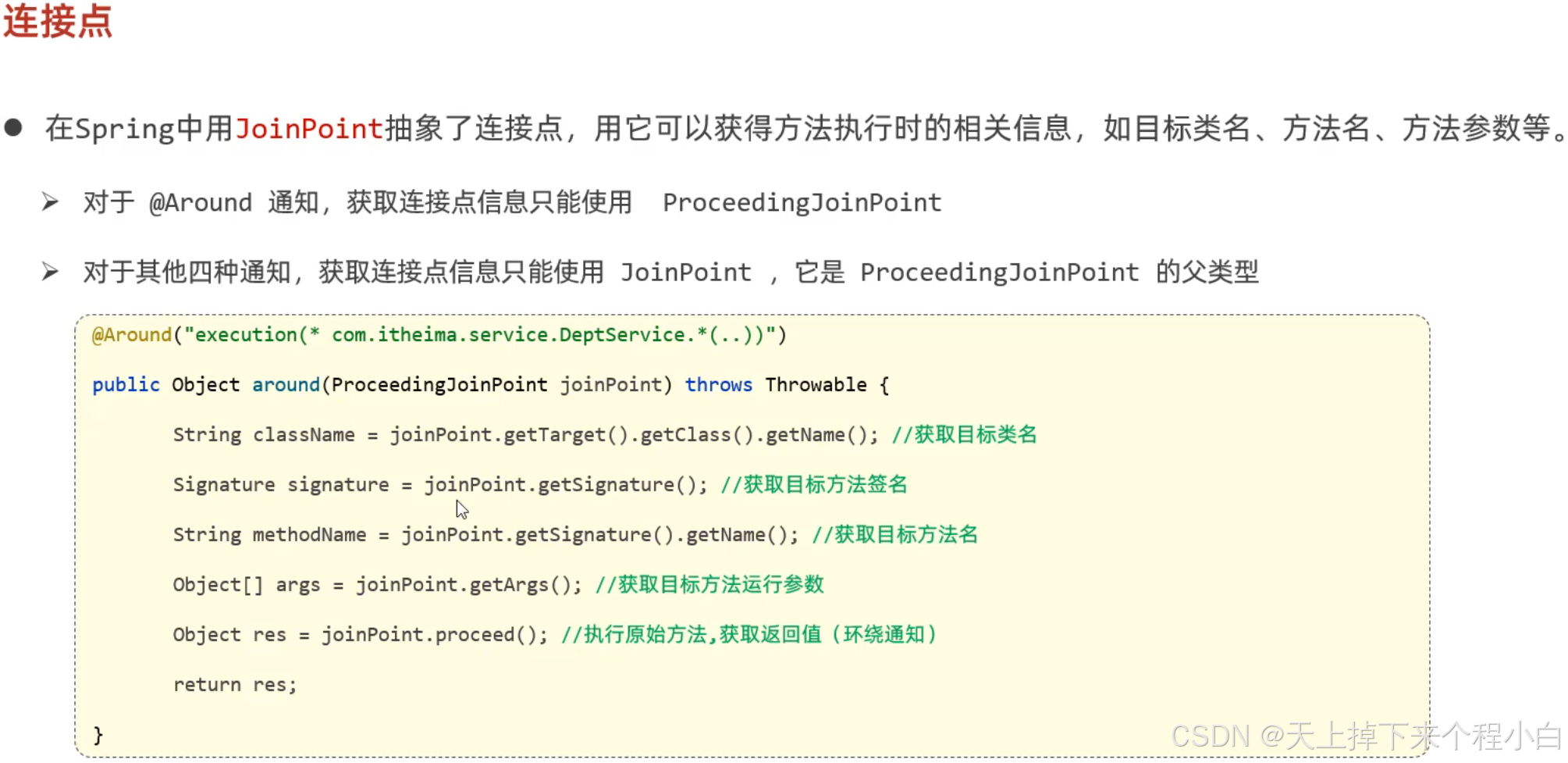
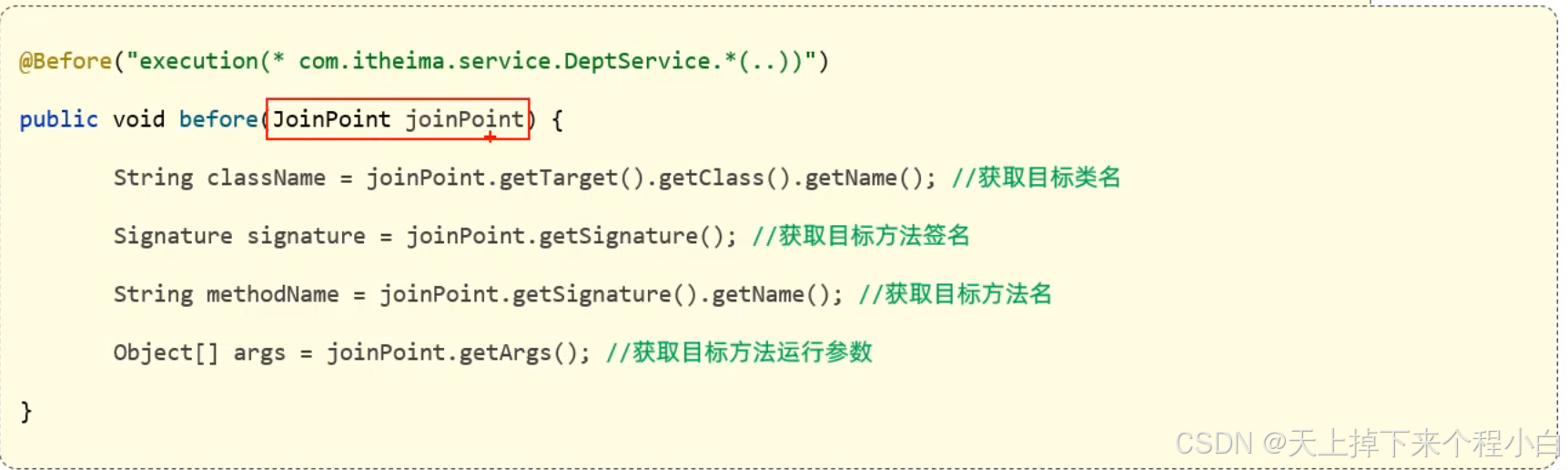
JoinPoint有两个,要使用org,aspectj.lang
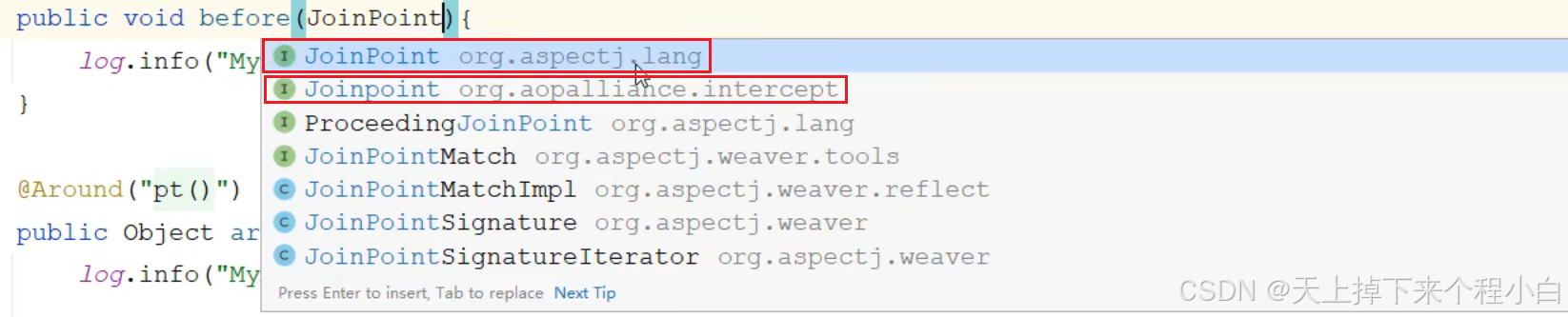
package com.gjw.aop;
import lombok.extern.slf4j.Slf4j;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
import java.util.Arrays;
/**
* 目标:掌握AOP中的连接点技术
* 所谓连接点,就是可以被AOP控制的方法,而在SpringAOP中,这个链接点又特指方法的执行
* 在Spring中用JoinPoint抽象了连接点,用它可以获得方法执行时的相关信息,如目标类名、方法名、方法参数等
* 对于@Around通知,获取连接点信息只能使用 ProceedingJoinPoint
* 对于其他四种通知,获取连接点信息只能使用JoinPoint,它是ProceedingJoinPoint的父类型
*/
@Slf4j
@Component
@Aspect
public class MyAspect8 {
@Pointcut("execution(* com.gjw.service.impl.DeptServiceImpl.*(..))")
public void pt(){}
@Around("pt()")
public Object around(ProceedingJoinPoint joinPoint) throws Throwable {
log.info("MyAspect8 around before......");
// 1. 获取 目标对象的类名
String className = joinPoint.getTarget().getClass().getName();
log.info("目标对象的类名:{}",className);
// 2. 获取 目标对象的方法名
String methodName = joinPoint.getSignature().getName();
log.info("目标对象的方法名:{}",methodName);
// 3. 获取 目标方法运行时传入的参数
Object[] args = joinPoint.getArgs();
log.info("目标方法运行时传入的参数:{}", Arrays.toString(args));
// 4. 放行 目标方法执行
Object result = joinPoint.proceed();
// 5. 获取 目标方法运行后的返回值
log.info("目标方法运行后的返回值:{}", result);
log.info("MyAspect8 around after");
return result;
}
}
Test中的测试代码
package com.gjw.springbootaopquickstart;
import com.gjw.pojo.Dept;
import com.gjw.service.DeptService;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.List;
@SpringBootTest
class SpringbootAopQuickstartApplicationTests {
@Autowired
private DeptService deptService;
@Test
void contextLoads() {
}
@Test
public void testAopDelete() {
deptService.deleteById(10);
}
@Test
public void testAopList() {
List<Dept> list = deptService.list();
System.out.println(list);
}
@Test
public void testAopGetById() {
Dept dept = deptService.getById(1);
System.out.println(dept);
}
}
执行testAopList()方法,执行结果如下:
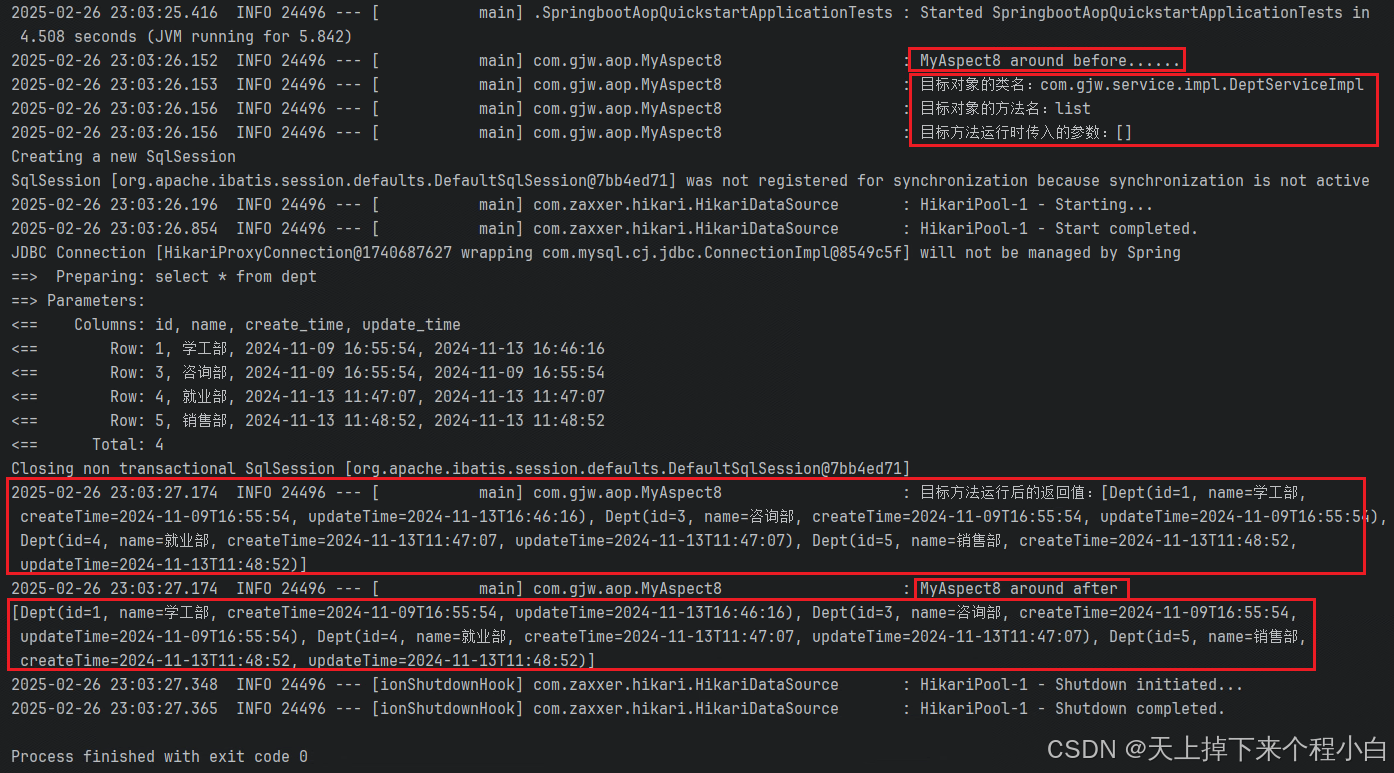
如果我们将result返回的结果改为null,则list方法(原始方法)就拿不到返回值了。返回null,就相当于原始方法执行完后,结果就丢了。因此要将result返回回去,设置通知方法的返回值类型为Object。
执行testAopDelete()方法,执行结果如下:
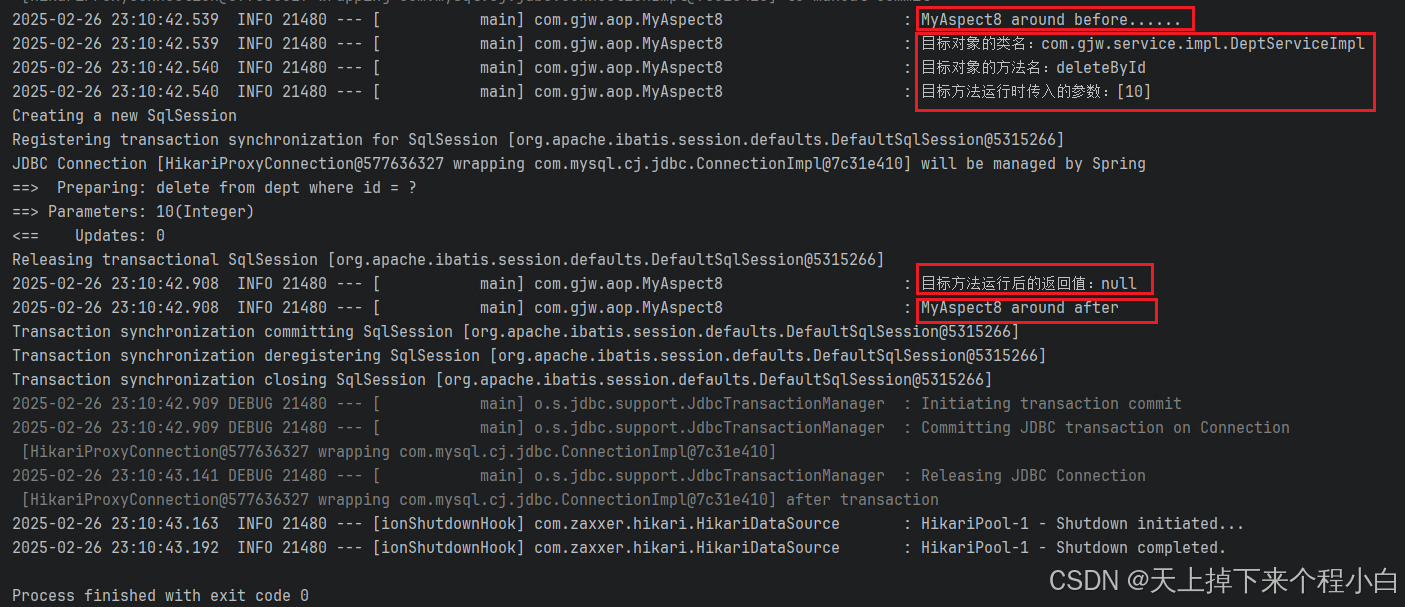
delete方法没有返回值。