前言:
目前java操作redis的客户端有jedis跟Lettuce。在springboot1.x系列中,其中使用的是jedis,
但是到了springboot2.x其中使用的是Lettuce。 因为我们的版本是springboot2.x系列,所以今天使用的是Lettuce。
关于jedis跟lettuce的区别:
Lettuce 和 Jedis 的定位都是Redis的client,所以他们当然可以直接连接redis server。
Jedis在实现上是直接连接的redis server,如果在多线程环境下是非线程安全的,这个时候只有使用连接池,
为每个Jedis实例增加物理连接
Lettuce的连接是基于Netty的,连接实例(StatefulRedisConnection)可以在多个线程间并发访问,
应为StatefulRedisConnection是线程安全的,所以一个连接实例(StatefulRedisConnection)就可以满足多线程环境下的并发访问,
当然这个也是可伸缩的设计,一个连接实例不够的情况也可以按需增加连接实例。
依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.1.3.RELEASE</version>
</dependency>
<!-- test单元测试用 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- redis依赖commons-pool 这个依赖一定要添加 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.6.2</version>
</dependency>
</dependencies>
application.yml配置
server:
port: 8989
spring:
redis:
host: 127.0.0.1
port: 6379
# 密码 没有则可以不填
password: 123456
# 如果使用的jedis 则将lettuce改成jedis即可
lettuce:
pool:
# 最大活跃链接数 默认8
max-active: 8
# 最大空闲连接数 默认8
max-idle: 8
# 最小空闲连接数 默认0
min-idle: 0
redis配置
接下来我们需要配置redis的key跟value的序列化方式,默认使用的JdkSerializationRedisSerializer 这样的会导致我们
通过redis desktop manager显示的我们key跟value的时候显示不是正常字符。 所以我们需要手动配置一下序列化方式 新建一个
config包,在其下新建一个RedisConfig.java 具体代码如下
/**
* @Auther: yukong
* @Date: 2018/8/17 14:58
* @Description: redis配置
*/
@Configuration
@AutoConfigureAfter(RedisAutoConfiguration.class)
public class RedisConfig {
/**
* 配置自定义redisTemplate
* @return
*/
@Bean
RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
//使用Jackson2JsonRedisSerializer来序列化和反序列化redis的value值
Jackson2JsonRedisSerializer serializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper mapper = new ObjectMapper();
mapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
mapper.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
serializer.setObjectMapper(mapper);
template.setValueSerializer(serializer);
//使用StringRedisSerializer来序列化和反序列化redis的key值
template.setKeySerializer(new StringRedisSerializer());
template.setHashKeySerializer(new StringRedisSerializer());
template.setHashValueSerializer(serializer);
template.afterPropertiesSet();
return template;
}
}
其中@Configuration 代表这个类是一个配置类,然后@AutoConfigureAfter(RedisAutoConfiguration.class) 是让我们这个配置类
在内置的配置类之后在配置,这样就保证我们的配置类生效,并且不会被覆盖配置。其中需要注意的就是方法名一定要
叫redisTemplate 因为@Bean注解是根据方法名配置这个bean的name的。
测试
package com.netcast.demo;
import com.netcast.demo.entity.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.test.context.junit4.SpringRunner;
/**
* @program: demo
* @description:
* @author: dchen
* @create: 2019-11-14 14:53
**/
@RunWith(SpringRunner.class)
@SpringBootTest
public class RedisTest {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void redisTest() {
// redis存储数据
String key = "name";
redisTemplate.opsForValue().set(key, "yukong");
// 获取数据
String value = (String) redisTemplate.opsForValue().get(key);
System.out.println("获取缓存中key为" + key + "的值为:" + value);
User user = new User();
user.setId("2");
user.setName("yukong");
user.setPhone("155662727272");
String userKey = "yukong";
redisTemplate.opsForValue().set(userKey, user);
User newUser = (User) redisTemplate.opsForValue().get(userKey);
System.out.println("获取缓存中key为" + userKey + "的值为:" + newUser);
}
}
结果:
中文成功显示,并且对象在redis以json方式存储,代表我们配置成功。
下列的就是Redis其它类型所对应的操作方式
opsForValue: 对应 String(字符串)
opsForZSet: 对应 ZSet(有序集合)
opsForHash: 对应 Hash(哈希)
opsForList: 对应 List(列表)
opsForSet: 对应 Set(集合)

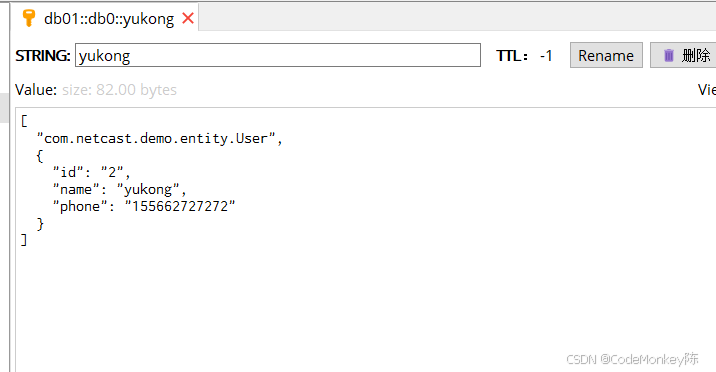