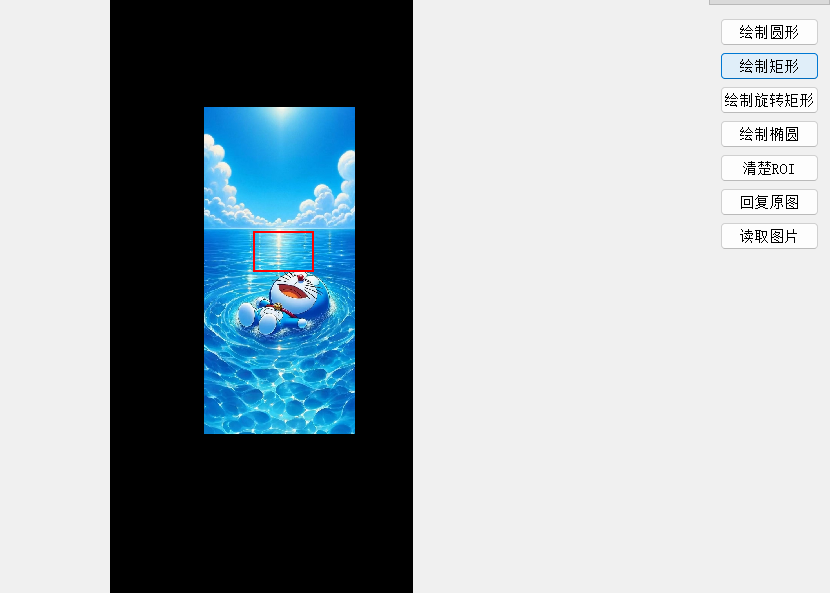
Halcon 操纵界面代码
#pragma once#include <QLabel>#include <halconcpp/HalconCpp.h>
#include <qtimer.h>
#include <qevent.h>
using namespace HalconCpp;#pragma execution_character_set("utf-8")class CHalconLabel : public QLabel
{Q_OBJECTpublic:CHalconLabel(QWidget* parent);~CHalconLabel();protected:void resizeEvent(QResizeEvent* ev); void wheelEvent(QWheelEvent* ev); void mousePressEvent(QMouseEvent* ev); void mouseReleaseEvent(QMouseEvent* ev); void mouseMoveEvent(QMouseEvent* ev); public:void SetID(); void SetPixelTracke(bool); void DisplayImage(HObject hDisplayImage); void ResetDisplayImage(); void DrawCircles(); void DrawRectangles(); void DrawRotateRectangles(); void DrawEllipses(); void ClearROI(); void DispalyImageROI(); void DisplayRegion(); HObject GetRegion();private:HTuple m_hLabelID; HTuple m_hHalconID = NULL; HObject m_drawnRegion; HObject ho_ImageZoom; HObject hCurrentImage; HTuple m_tMouseDownRow, m_tMouseDownCol; bool m_bIsMove; bool m_bIsDrawROI; HObject ho_Image;};
#include "CHalconLabel.h"CHalconLabel::CHalconLabel(QWidget* parent): QLabel(parent), m_bIsMove(false), m_bIsDrawROI(false)
{GenEmptyObj(&hCurrentImage);GenEmptyObj(&m_drawnRegion);this->setAlignment(Qt::AlignTop | Qt::AlignHCenter);this->setStyleSheet("color: red;");}CHalconLabel::~CHalconLabel()
{
}void CHalconLabel::resizeEvent(QResizeEvent * ev)
{if (m_hHalconID != NULL){SetSystem("flush_graphic", "false");ClearWindow(m_hHalconID);DetachBackgroundFromWindow(m_hHalconID);int labelWidth = this->width(); int labelHeight = this->height();HTuple imgWidth, imgHeight; HTuple m_scaledWidth, m_scaledHeight; HTuple m_hvScaledRate; GetImageSize(hCurrentImage, &imgWidth, &imgHeight);TupleMin2(1.0 * labelWidth / imgWidth, 1.0 * labelHeight / imgHeight, &m_hvScaledRate);ZoomImageFactor(hCurrentImage, &ho_ImageZoom, m_hvScaledRate, m_hvScaledRate, "constant");GetImageSize(ho_ImageZoom, &m_scaledWidth, &m_scaledHeight);if (1.0 * labelWidth / imgWidth < 1.0 * labelHeight / imgHeight){SetWindowExtents(m_hHalconID, labelHeight / 2.0 - m_scaledHeight / 2.0, 0, labelWidth, m_scaledHeight);}else{SetWindowExtents(m_hHalconID, 0, labelWidth / 2.0 - m_scaledWidth / 2.0, m_scaledWidth, labelHeight);}SetPart(m_hHalconID, 0, 0, imgHeight - 1, imgWidth - 1);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);SetSystem("flush_graphic", "true");DetachBackgroundFromWindow(m_hHalconID);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);DispObj(hCurrentImage, m_hHalconID);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);DispObj(m_drawnRegion, m_hHalconID); }
}void CHalconLabel::wheelEvent(QWheelEvent* ev)
{double Zoom; HTuple mouseRow, mouseCol, Button;HTuple startRowBf, startColBf, endRowBf, endColBf, Ht, Wt, startRowAft, startColAft, endRowAft, endColAft;if (ev->delta() > 0){Zoom = 2.0;}else{Zoom = 1 / 2.0;}HTuple hv_Exception, hv_ErrMsg;try{GetMposition(m_hHalconID, &mouseRow, &mouseCol, &Button);}catch (HException& HDevExpDefaultException){return;}GetPart(m_hHalconID, &startRowBf, &startColBf, &endRowBf, &endColBf);Ht = endRowBf - startRowBf;Wt = endColBf - startColBf;if (Ht * Wt < 20000 * 20000 || Zoom == 2.0){startRowAft = mouseRow - ((mouseRow - startRowBf) / Zoom);startColAft = mouseCol - ((mouseCol - startColBf) / Zoom);endRowAft = startRowAft + (Ht / Zoom);endColAft = startColAft + (Wt / Zoom);if (endRowAft - startRowAft < 2){return;}if (m_hHalconID != NULL){DetachBackgroundFromWindow(m_hHalconID);}SetPart(m_hHalconID, startRowAft, startColAft, endRowAft, endColAft);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);}AttachBackgroundToWindow(hCurrentImage, m_hHalconID);DispObj(m_drawnRegion, m_hHalconID);
}void CHalconLabel::mousePressEvent(QMouseEvent* ev)
{HTuple mouseRow, mouseCol, Button;try{GetMposition(m_hHalconID, &mouseRow, &mouseCol, &Button);}catch (HException){return;}m_tMouseDownRow = mouseRow;m_tMouseDownCol = mouseCol;m_bIsMove = true;
}void CHalconLabel::mouseReleaseEvent(QMouseEvent* ev)
{m_bIsMove = false;}void CHalconLabel::mouseMoveEvent(QMouseEvent* ev)
{if (m_bIsDrawROI) {this->setCursor(Qt::ArrowCursor); return;}HTuple startRowBf, startColBf, endRowBf, endColBf, mouseRow, mouseCol, Button;try{SetCheck("~give_error"); GetMposition(m_hHalconID, &mouseRow, &mouseCol, &Button);if (mouseCol.Length() <= 0 || mouseRow.Length() < 0){return;}SetCheck("give_error");}catch (HException){return;}if (m_bIsMove){this->setCursor(Qt::PointingHandCursor); double RowMove = mouseRow[0].D() - m_tMouseDownRow[0].D();double ColMove = mouseCol[0].D() - m_tMouseDownCol[0].D();GetPart(m_hHalconID, &startRowBf, &startColBf, &endRowBf, &endColBf);if (m_hHalconID != NULL){DetachBackgroundFromWindow(m_hHalconID);}SetPart(m_hHalconID, startRowBf - RowMove, startColBf - ColMove, endRowBf - RowMove, endColBf - ColMove);SetCheck("~give_error");AttachBackgroundToWindow(hCurrentImage, m_hHalconID);SetCheck("give_error");}else {this->setCursor(Qt::ArrowCursor); HTuple pointGray;try{SetCheck("~give_error"); GetGrayval(hCurrentImage, mouseRow, mouseCol, &pointGray);if (mouseCol.Length() <= 0 || pointGray[0].D() < 0){return;}SetCheck("give_error"); }catch (HException){return;}}AttachBackgroundToWindow(hCurrentImage, m_hHalconID);DispObj(m_drawnRegion, m_hHalconID);
}void CHalconLabel::DrawCircles()
{m_bIsDrawROI = true;HObject currentRegion;HTuple Row, Column, Radius;DrawCircle(m_hHalconID, &Row, &Column, &Radius);GenCircle(¤tRegion, Row, Column, Radius);Union2(m_drawnRegion, currentRegion, &m_drawnRegion);SetColor(m_hHalconID, "red");SetDraw(m_hHalconID, "margin");SetLineWidth(m_hHalconID, 2);DispObj(m_drawnRegion, m_hHalconID);m_bIsDrawROI = false;
}void CHalconLabel::DrawRectangles()
{m_bIsDrawROI = true;HObject currentRegion;HTuple R1, C1, R2, C2;DrawRectangle1(m_hHalconID, &R1, &C1, &R2, &C2);GenRectangle1(¤tRegion, R1, C1, R2, C2);Union2(m_drawnRegion, currentRegion, &m_drawnRegion);SetColor(m_hHalconID, "red");SetDraw(m_hHalconID, "margin");SetLineWidth(m_hHalconID, 2);DispObj(m_drawnRegion, m_hHalconID);m_bIsDrawROI = false;
}void CHalconLabel::DrawRotateRectangles()
{m_bIsDrawROI = true;HObject currentRegion;HTuple Row, Column, Phi, Length1, Length2;DrawRectangle2(m_hHalconID, &Row, &Column, &Phi, &Length1, &Length2);GenRectangle2(¤tRegion, Row, Column, Phi, Length1, Length2);Union2(m_drawnRegion, currentRegion, &m_drawnRegion);SetColor(m_hHalconID, "red");SetDraw(m_hHalconID, "margin");SetLineWidth(m_hHalconID, 2);DispObj(m_drawnRegion, m_hHalconID);m_bIsDrawROI = false;
}void CHalconLabel::DrawEllipses()
{m_bIsDrawROI = true;HObject currentRegion;HTuple Row, Column, Phi, Radius1, Radius2;DrawEllipse(m_hHalconID, &Row, &Column, &Phi, &Radius1, &Radius2);GenEllipse(¤tRegion, Row, Column, Phi, Radius1, Radius2);Union2(m_drawnRegion, currentRegion, &m_drawnRegion);SetColor(m_hHalconID, "red");SetDraw(m_hHalconID, "margin");SetLineWidth(m_hHalconID, 2);DispObj(m_drawnRegion, m_hHalconID);m_bIsDrawROI = false;
}void CHalconLabel::ClearROI()
{GenEmptyRegion(&m_drawnRegion);
}void CHalconLabel::DispalyImageROI()
{DisplayImage(hCurrentImage);HObject emptyRegion;HTuple isEqual;GenEmptyRegion(&emptyRegion);TestEqualRegion(emptyRegion, m_drawnRegion, &isEqual);if (isEqual == 0) {DispObj(m_drawnRegion, m_hHalconID);}
}void CHalconLabel::DisplayRegion()
{HObject image;HObject emptyRegion;HTuple isEqual;GenEmptyRegion(&emptyRegion);TestEqualRegion(emptyRegion, m_drawnRegion, &isEqual);if (isEqual != 0) {return;}ReduceDomain(hCurrentImage, m_drawnRegion, &image);ClearWindow(m_hHalconID);DisplayImage(image);
}HObject CHalconLabel::GetRegion()
{return m_drawnRegion;
}void CHalconLabel::SetID()
{if (m_hHalconID == NULL) {SetWindowAttr("background_color", "black"); m_hLabelID = (Hlong)this->winId();OpenWindow(0, 0, this->width(), this->height(), m_hLabelID, "visible", "", &m_hHalconID);}
}void CHalconLabel::SetPixelTracke(bool ret)
{this->setMouseTracking(ret);}void CHalconLabel::DisplayImage(HObject hDisplayImage)
{CopyImage(hDisplayImage, &hCurrentImage);ClearWindow(m_hHalconID);DetachBackgroundFromWindow(m_hHalconID);int labelWidth = this->width(); int labelHeight = this->height();HTuple imgWidth, imgHeight; HTuple m_scaledWidth, m_scaledHeight; HTuple m_hvScaledRate; GetImageSize(hCurrentImage, &imgWidth, &imgHeight);TupleMin2(1.0 * labelWidth / imgWidth, 1.0 * labelHeight / imgHeight, &m_hvScaledRate);ZoomImageFactor(hCurrentImage, &ho_ImageZoom, m_hvScaledRate, m_hvScaledRate, "constant");GetImageSize(ho_ImageZoom, &m_scaledWidth, &m_scaledHeight);if (1.0 * labelWidth / imgWidth < 1.0 * labelHeight / imgHeight){SetWindowExtents(m_hHalconID, labelHeight / 2.0 - m_scaledHeight / 2.0, 0, labelWidth, m_scaledHeight);}else{SetWindowExtents(m_hHalconID, 0, labelWidth / 2.0 - m_scaledWidth / 2.0, m_scaledWidth, labelHeight);}SetPart(m_hHalconID, 0, 0, imgHeight - 1, imgWidth - 1);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);}void CHalconLabel::ResetDisplayImage()
{ClearWindow(m_hHalconID);DetachBackgroundFromWindow(m_hHalconID);int labelWidth = this->width(); int labelHeight = this->height();HTuple imgWidth, imgHeight; HTuple m_scaledWidth, m_scaledHeight; HTuple m_hvScaledRate; GetImageSize(hCurrentImage, &imgWidth, &imgHeight);TupleMin2(1.0 * labelWidth / imgWidth, 1.0 * labelHeight / imgHeight, &m_hvScaledRate);ZoomImageFactor(hCurrentImage, &ho_ImageZoom, m_hvScaledRate, m_hvScaledRate, "constant");GetImageSize(ho_ImageZoom, &m_scaledWidth, &m_scaledHeight);if (1.0 * labelWidth / imgWidth < 1.0 * labelHeight / imgHeight){SetWindowExtents(m_hHalconID, labelHeight / 2.0 - m_scaledHeight / 2.0, 0, labelWidth, m_scaledHeight);}else{SetWindowExtents(m_hHalconID, 0, labelWidth / 2.0 - m_scaledWidth / 2.0, m_scaledWidth, labelHeight);}SetPart(m_hHalconID, 0, 0, imgHeight - 1, imgWidth - 1);AttachBackgroundToWindow(hCurrentImage, m_hHalconID);
}
窗口代码
#pragma once#include <QtWidgets/QMainWindow>#include "CHalconLabel.h"#include "ui_HalconMain.h"
#include <qpushbutton.h>
#include <qfiledialog.h>class HalconMain : public QMainWindow
{Q_OBJECTpublic:HalconMain(QWidget *parent = nullptr);~HalconMain();public:void InitWidget();private slots:void on_readImageBtn();void on_drawCircleBtn();void on_drawRectangleBtn();void on_drawRotateBtn();void on_drawEllipseBtn();void on_clearROIBtn();void on_resetImageBtn();private:Ui::HalconMainClass ui;CHalconLabel* displayLabel;
};
HalconMain::HalconMain(QWidget *parent): QMainWindow(parent)
{ui.setupUi(this);InitWidget();connect(ui.pb_readImg, &QPushButton::clicked, this, &HalconMain::on_readImageBtn);connect(ui.pb_drawcircle, &QPushButton::clicked, this, &HalconMain::on_drawCircleBtn);connect(ui.pb_drawrectangle, &QPushButton::clicked, this, &HalconMain::on_drawRectangleBtn);connect(ui.pb_clearroi, &QPushButton::clicked, this, &HalconMain::on_clearROIBtn);connect(ui.pb_recoverimg, &QPushButton::clicked, this, &HalconMain::on_resetImageBtn);}HalconMain::~HalconMain()
{
}void HalconMain::InitWidget()
{displayLabel = new CHalconLabel(this);ui.layout->addWidget(displayLabel);
}void HalconMain::on_drawCircleBtn()
{displayLabel->DrawCircles();
}void HalconMain::on_drawRectangleBtn()
{displayLabel->DrawRectangles();
}void HalconMain::on_drawRotateBtn()
{}void HalconMain::on_drawEllipseBtn()
{
}void HalconMain::on_clearROIBtn()
{displayLabel->ClearROI();displayLabel->DispalyImageROI();
}void HalconMain::on_resetImageBtn()
{displayLabel->DispalyImageROI();
}void HalconMain::on_readImageBtn()
{HObject hImage;displayLabel->SetID(); QString fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), ".", tr("Image Files (*.png *.jpg *.bmp)"));if (fileName.isEmpty()) {return;}HTuple hFileName(fileName.toStdString().c_str());ReadImage(&hImage, hFileName);displayLabel->DisplayImage(hImage);displayLabel->SetPixelTracke(false);
}
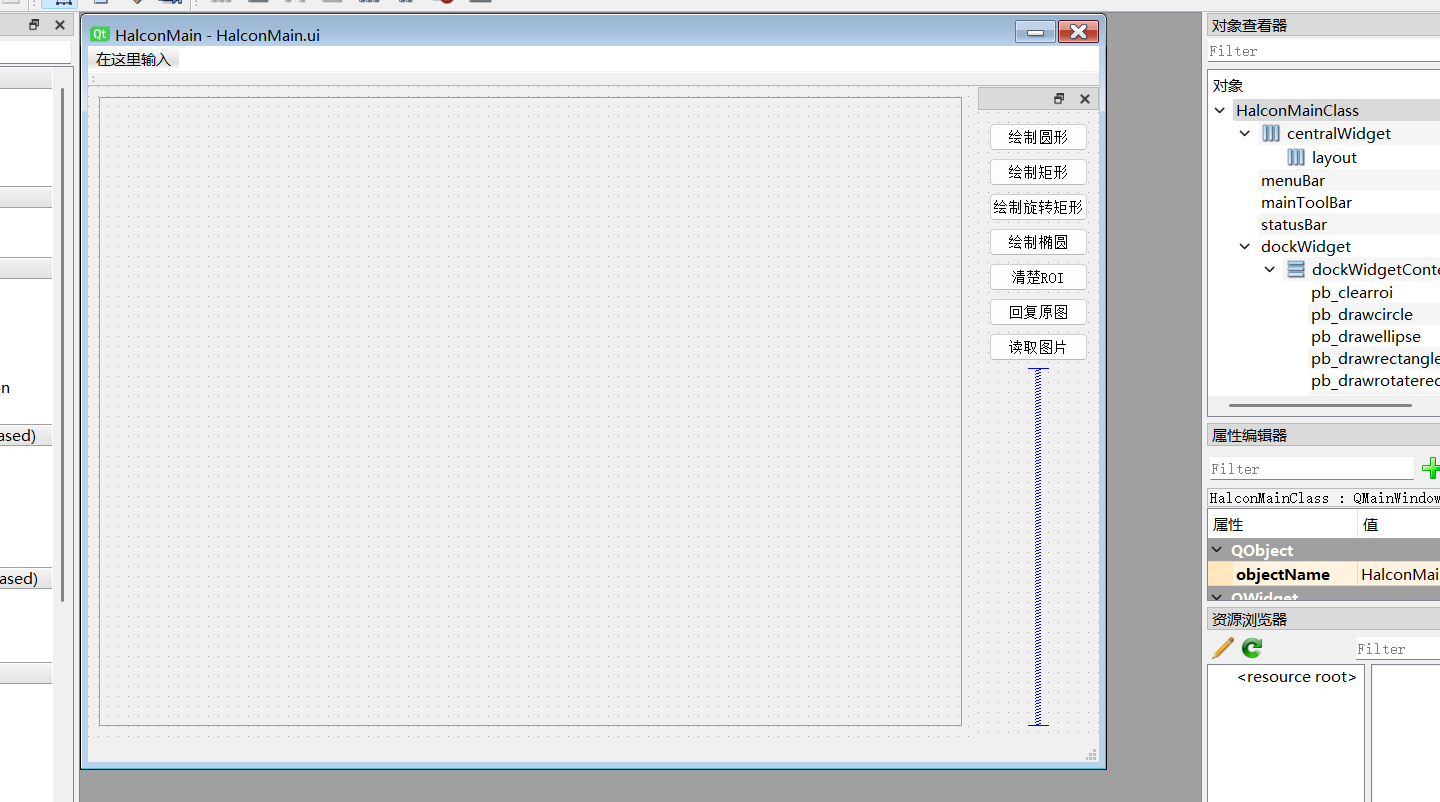