C++学习之云盘项目总结
目录
1.知识点总结
2.bug修改
3.客户端显示文件列表协议
4.nginx中Location
5.文件列表代码分析1
6.文件列表代码处理流程代码
7.文件下载流程处理
8.项目架构和功能点
9.项目2知识点提炼
10.项目2 知识点提炼2
11.fastdfs存储节点的反向代理
12.QT中tcp通信的服务端代码实现
13.套接字客户端实现
14.QT线程中的使用方式1
15.QT中线程中第二种使用方式
16.第二种线程方式注意事项
17.多线程实例程序
1.知识点总结
Qt
中相关的布局类
1. QHBoxLayout -
水平布局
2. QVBoxLayout -
垂直布局
3. QGridLayout -
网格布局
2.
套接字通信
1. TCP
通信
/* QT
中的布局操作
1.
布局中可以添加布局
2.
布局中可以添加插入窗口
3.
布局单独是不能显示的
4.
需要将布局设置给窗口
*/
void
QBoxLayout::addSpacing
(
int
size
)
-
//
添加固定的间隙
void
QBoxLayout::addStretch
(
int
stretch
=
0
)
-
//
添加弹簧
//
在布局的最后添加新窗口
void
QBoxLayout::addWidget
(
QWidget
*
widget
,
int
stretch
=
0
,
Qt::Alignment alignment
=
Qt::Alignment
())
//
在指定的位置插入新窗口
void
QBoxLayout::insertWidget
(
int
index
,
QWidget
*
widget
,
int
stretch
=
0
,
Qt::Alignment
alignment
=
Qt::Alignment
())
-
参数
index
:
创建
widget
插入到
index
窗口之前
//
从布局中删除窗口
void
QLayout::removeWidget
(
QWidget
*
widget
)
//
将布局设置给窗口
void
QWidget::setLayout
(
QLayout
*
layout
)
//
布局窗口的个数
int
QLayout::count
()
const
19
for
(
int
i
=
0
;
i
<
layout
->
count
();
++
i
)
{
//
取出每一个窗口对象
QLayoutItem
*
child
=
layout
->
takeAt
(
i
);
QWidget
*
wg
=
child
->
widget
();
wg
->
setParent
(
NULL
);
layout
->
removeWidget
(
wg
);
delete
child
;
}
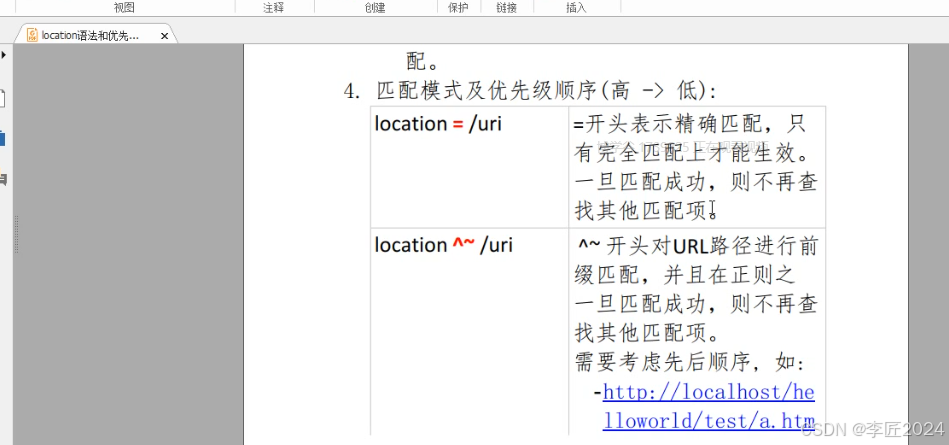
2.bug修改
套接字通信
1. TCP
通信
/* QT
中的布局操作
1.
布局中可以添加布局
2.
布局中可以添加插入窗口
3.
布局单独是不能显示的
4.
需要将布局设置给窗口
*/
void
QBoxLayout::addSpacing
(
int
size
)
-
//
添加固定的间隙
void
QBoxLayout::addStretch
(
int
stretch
=
0
)
-
//
添加弹簧
//
在布局的最后添加新窗口
void
QBoxLayout::addWidget
(
QWidget
*
widget
,
int
stretch
=
0
,
Qt::Alignment alignment
=
Qt::Alignment
())
//
在指定的位置插入新窗口
void
QBoxLayout::insertWidget
(
int
index
,
QWidget
*
widget
,
int
stretch
=
0
,
Qt::Alignment
alignment
=
Qt::Alignment
())
-
参数
index
:
创建
widget
插入到
index
窗口之前
//
从布局中删除窗口
void
QLayout::removeWidget
(
QWidget
*
widget
)
//
将布局设置给窗口
void
QWidget::setLayout
(
QLayout
*
layout
)
//
布局窗口的个数
int
QLayout::count
()
const
19
for
(
int
i
=
0
;
i
<
layout
->
count
();
++
i
)
{
//
取出每一个窗口对象
QLayoutItem
*
child
=
layout
->
takeAt
(
i
);
QWidget
*
wg
=
child
->
widget
();
wg
->
setParent
(
NULL
);
layout
->
removeWidget
(
wg
);
delete
child
;
}
9
// QTcpServer -
监听
// TcpSocket -
通信
//
服务器端
:
/*
1
2
3
4
2. UDP
通信
1.
创建一监听的
QTcpServer
监听对象
2.
监听
3.
等待并接受连接请求
4.
通信
5.
关闭套接字
/
释放对象
*/
QTcpServer::QTcpServer
(
QObject
*
parent
=
Q_NULLPTR
)
bool
QTcpServer::listen
(
const
QHostAddress
&
address
=
QHostAddress::Any
,
quint16 port
=
0
)
// QTcpServer
检测到有新连接
,
会发出该信号
[
signal
]
void
QTcpServer::newConnection
()
//
接受一个客户端的连接请求
QTcpSocket
*
QTcpServer::nextPendingConnection
()
-
返回值
:
和已连接的客户端通信的对象
//
通信过程
//
发送数据
-
主动操作
qint64
QIODevice::write
(
const
QByteArray
&
byteArray
)
//
接收数据
-
被动
//
需要知道数据什么时候到达了
,
通过信号槽机制
[
signal
]
void
QIODevice::readyRead
()
/*
客户端
:
1.
创建通信的套接字对象
QTcpSocket
2.
连接服务器
3.
通信
4.
关闭套接字
/
释放对象
*/
QTcpSocket::QTcpSocket
(
QObject
*
parent
=
Q_NULLPTR
)
void
QAbstractSocket::connectToHost
(
const
QString
&
hostName
,
quint16 port
,
OpenMode
openMode
=
ReadWrite
,
NetworkLayerProtocol protocol
=
AnyIPProtocol
)
void
QAbstractSocket::connectToHost
(
const
QHostAddress
&
address
,
quint16 port
,
OpenMode
openMode
=
ReadWrite
)
35
// QUdpSocket
/*
通信流程
1.
创建
QUdpSocket
2.
绑定
IP
和端口
(
如果当前程序不需要接收数据
,
可以省略此步骤
)
3.
通信
-
发送数据
-
接收数据
4.
释放资源
*/
QUdpSocket
(
QObject
*
parent
=
Q_NULLPTR
)
//
发送报文
qint64
QUdpSocket::writeDatagram
(
const
char *
data
,
qint64 size
,
const
QHostAddress
&
address
,
quint16 port
)
13
14
qint64
QUdpSocket::writeDatagram
(
const
QByteArray
&
datagram
,
const
QHostAddress
&
host
,
quint16 port
)
-
参数
datagram
:
发送的数据
-
参数
host
:
接收数据的主机的
IP
地址
-
参数
port
:
接收数据的主机绑定的端口
//
获取要接收的报文的长度
qint64
QUdpSocket::pendingDatagramSize
()
const
//
接收报文
qint64
QUdpSocket::readDatagram
(
char *
data
,
qint64 maxSize
,
QHostAddress
*
address
=
Q_NULLPTR
,
quint16
*
port
=
Q_NULLPTR
)
22
//
应用程序
1
QUdpSocket
*
udp
=
new
QUdpSocket
(
this
);
//
接收数据
udp
->
bind
(
IP
,
8989
);
//
给
app2
发送数据
udp
->
writeDatagram
(
"hell, app2"
,
QHostAddress
(
"192.168.1.22"
),
8000
);
connect
(
udp
,
QUdpSocket::readyRead
,
this
, [
=
]()
{
//
获取要接收的数据长度
qint64 size
=
udp
->
pendingDatagramSize
()
QByteArray array
(
size
,
0
);
udp
->
readDatagram
(
array
.
data
(),
size
);
});
//
应用程序
2
QUdpSocket
*
udp
=
new
QUdpSocket
(
this
);
//
接收数据
udp
->
bind
(
IP
,
8000
);
//
给
app1
发送数据
udp
->
writeDatagram
(
"hell, app1"
,
QHostAddress
(
"192.168.1.23"
),
8989
);
connect
(
udp
,
QUdpSocket::readyRead
,
this
, [
=
]()
{
//
获取要接收的数据长度
qint64 size
=
udp
->
pendingDatagramSize
()
QByteArray array
(
size
,
0
);
udp
->
readDatagram
(
array
.
data
(),
size
);
});
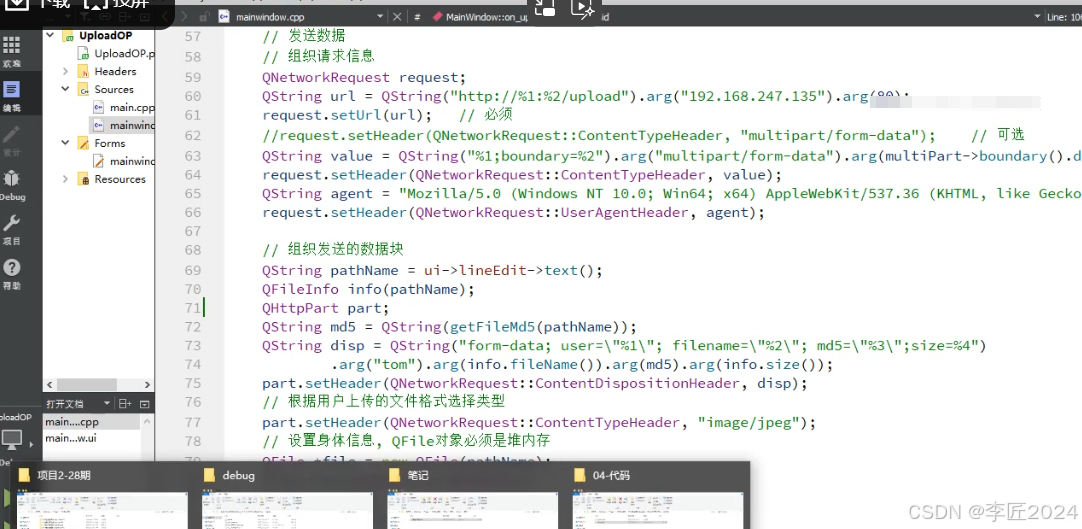