Redis基础学习
目录
Redis命令
通用命令
String
Key的顶层格式
Hash
List
Set
SortedSet
Redis命令
通用命令
想知道某个命令怎么用
1.可以在官网学习用法
https://www.redis.net.cn/order/
2. 使用 help+命令
String
Key的顶层格式
Hash
List
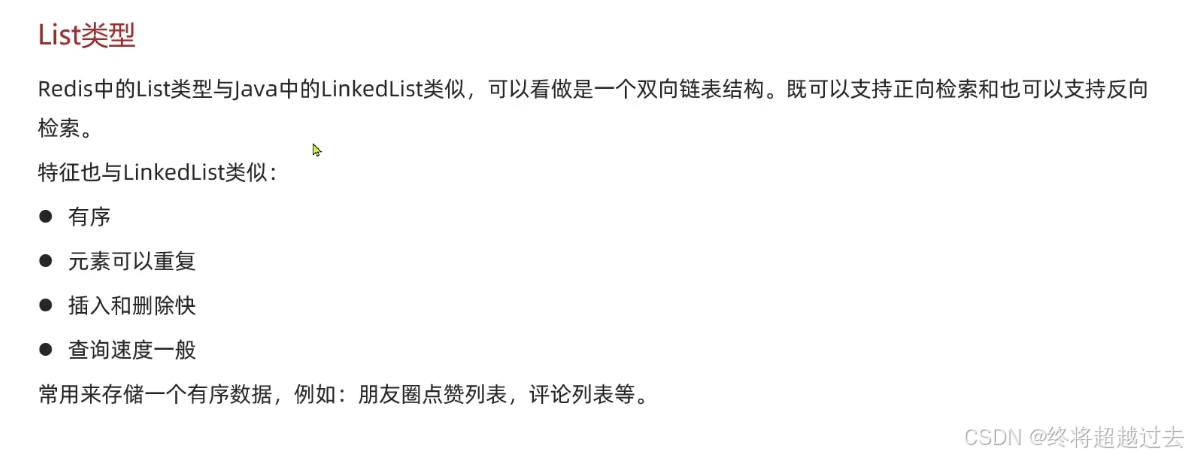
Set
SortedSet
在IDEA使用Jedis操作Redis
常规使用
引入依赖
我的pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.leo</groupId>
<artifactId>jedis-work</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<name>jedis-work</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<junit.jupiter.version>5.7.0</junit.jupiter.version>
</properties>
<dependencies>
<!--jedis-->
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.7.0</version>
</dependency>
<!--单元测试-->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<includes>
<include>**/*Test.java</include>
<include>**/*Tests.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
</project>
我的测试
package com.leo.test;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import redis.clients.jedis.Jedis;
import org.junit.jupiter.api.Test;
public class TestRedis {
private Jedis jedis;
@BeforeEach
void setUp() {
// 1.建立连接
jedis = new Jedis("192.168.**.**", 6379); //根据自己的ip
// 2.设置密码
jedis.auth("***"); 自己的密码
// 3.选择库
jedis.select(0);
}
@Test
void testString() {
// 存入数据
String result = jedis.set("name2", "大哥");
System.out.println("result = " + result);
// 获取数据
String name = jedis.get("name2");
System.out.println("name = " + name);
}
@AfterEach
void tearDown() {
if (jedis != null) {
jedis.close();
}
}
}
Jedis的连接池
1.配置Jedis连接池工具类
package Utils;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
public class JedisConnectionFactory {
private static final JedisPool jedisPool;
static {
//配置连接池
JedisPoolConfig poolConfig = new JedisPoolConfig();
poolConfig.setMaxTotal(8);
poolConfig.setMaxIdle(8);
poolConfig.setMinIdle(0);
poolConfig.setMaxWaitMillis(1000);
//创建连接池对象
jedisPool = new JedisPool(poolConfig,
"192.168.88.134",6379,1000,"123321");
}
public static Jedis getJedis(){
return jedisPool.getResource();
}
}
建立连接使用工具类
package com.leo.test;
import Utils.JedisConnectionFactory;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import redis.clients.jedis.Jedis;
import org.junit.jupiter.api.Test;
import redis.clients.jedis.JedisFactory;
import redis.clients.jedis.JedisPool;
public class TestRedis {
private Jedis jedis;
@BeforeEach
void setUp() {
// 1.建立连接
// 方式1
// jedis = new Jedis("192.168.88.134", 6379);
// 方式2
jedis= JedisConnectionFactory.getJedis();
// 2.设置密码
jedis.auth("123321");
// 3.选择库
jedis.select(0);
}
@Test
void testString() {
// 存入数据
String result = jedis.set("name3", "小弟");
System.out.println("result = " + result);
// 获取数据
String name = jedis.get("name3");
System.out.println("name = " + name);
}
@AfterEach
void tearDown() {
if (jedis != null) {
jedis.close();
}
}
}