Spring Boot 整合 Servlet三大组件(Servlet / Filter / Listene)
Spring Boot 整合 “Servlet三大组件“ ( Servlet / Filter / Listene )
目录如下: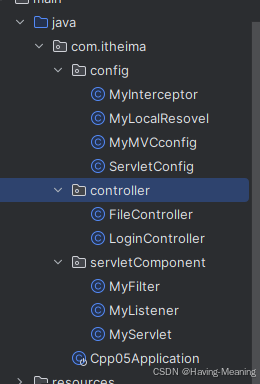
pom.xml配置
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.4.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>cpp05</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>cpp05</name>
<description>cpp05</description>
<url/>
<licenses>
<license/>
</licenses>
<developers>
<developer/>
</developers>
<scm>
<connection/>
<developerConnection/>
<tag/>
<url/>
</scm>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- 进行文件下载的工具依赖 -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
</dependency>
<dependency>
<groupId>jakarta.servlet</groupId>
<artifactId>jakarta.servlet-api</artifactId>
<version>5.0.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
</plugins>
</build>
</project>
ServletConfig 代码如下:
package com.itheima.config;
import com.itheima.servletComponent.MyFilter;
import com.itheima.servletComponent.MyServlet;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.Arrays;
@Configuration //将该类标记为"配置类"
public class ServletConfig { //Serlvet配置类
@Autowired
private MyServlet myServlet;
@Bean //将给方法的返回值对象加入到IOC容器中
//在SpringBoot中注册XxxRegistrationBean组件/对象
public ServletRegistrationBean getServlet() {
/*
/myServlet 这个请求映射到对应的Servlet对象上
*/
ServletRegistrationBean servletRegistrationBean =
new ServletRegistrationBean(myServlet,"/myServlet");
return servletRegistrationBean;
}
@Autowired
private MyFilter myFilter;
@Bean
public FilterRegistrationBean getFilter() { //将Filter组件加入到IOC容器中
FilterRegistrationBean filterRegistrationBean = new FilterRegistrationBean(myFilter);
//设置过滤器要过滤的url路径
filterRegistrationBean.setUrlPatterns(Arrays.asList("/toLoginPage","/myFilter"));
return filterRegistrationBean;
}
}
MyFilter 代码如下:
package com.itheima.servletComponent;
import jakarta.servlet.*;
import org.springframework.stereotype.Component;
import java.io.IOException;
@Component //加入到IOC容器中
public class MyFilter implements Filter { //实现 Filter/过滤器接口
@Override
public void init(FilterConfig filterConfig) throws ServletException {
Filter.super.init(filterConfig);
}
/**
* doFilter()方法在“请求处理之前”被执行,就是一个url请求,想到达doFilter()方法,再到达对应的Servlet组件的方法
*/
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
//对url请求进行过滤,url请求先在doFilter()方法中拦截,如被放行,才会接着去执行Servlet中的方法
System.out.println("hello MyFilter");
//将请求和响应对象传递给过滤器链中的下一个实体,这个实体可以是一个过滤器,或者是最终的Servlet或JSP页面
filterChain.doFilter(servletRequest, servletResponse);
}
@Override
public void destroy() {
Filter.super.destroy();
} //自定义“过滤器”/Filter类
}
MyListener
package com.itheima.servletComponent;
import jakarta.servlet.ServletContextEvent;
import jakarta.servlet.ServletContextListener;
import jakarta.servlet.annotation.WebListener;
import org.springframework.stereotype.Component;
//通过注解的方式来在SpringBoot中“整合Listener” ( 就是通过注解的方式来SpringBoot中能使用Listener )
//在web应用程序启动时会执行 contextInitialized()方法 , web应用程序结束/销毁后会执行contextDestroyed()方法
@WebListener
public class MyListener implements ServletContextListener { //自定义的“监听器”/Listener 对象
/*
* contextInitialized()方法是Web应用程序启动时被执行。
* 该方法的主要用途是对系统的全局变量、配置参数等进行初始化,确保系统在正常运行之前处于一个良好的状态
*/
@Override
public void contextInitialized(ServletContextEvent sce) { //该方法子啊web应用程序启动时被执行
System.out.println("contextInitialized.....(annotaion)");
}
/**
* contextDestroyed()方法在web应用程序的"生命周期结束"时/ web应用程序“销毁”时被执行
*/
@Override
public void contextDestroyed(ServletContextEvent sce) { //该方法在web应用程序的"生命周期结束"时被执行
System.out.println("contextInitialized.....(annotaion)");
}
}
MyServlet
package com.itheima.servletComponent;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import org.springframework.stereotype.Component;
import java.io.IOException;
/**
* SpringBoot中通过“注册组件”的方式来整合Servlet三大组件
* 即通过创建XxxRegistrationBean对象并将其加入到IOC容器中的方式来在SpringBoot中整合Servlet的三大组件
*/
@Component //加入到IOC容器中
public class MyServlet extends HttpServlet { //
/*
doGet()方法
*/
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doPost(request, response);
}
/*
dopost方法
*/
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//当url访问该Servlet中的doPost()方法会执行方法体中的代码
//客户端(如浏览器)向Servlet发送请求并收到响应时,它会看到响应的内容是 "hello MyServlet" 这个字符串。
response.getWriter().write("hello MyServlet");
}
}
运行结果如下:
访问http://localhost:8084/myServlet
访问http://localhost:8084/toLoginPage
监听: