class time_show:
def __init__(self):
self.__total_time = int(time.time())
self.__current_hours = (self.__total_time // 3600) % 24 % 365 + 8
self.__current_minute = self.__total_time % 3600 // 60
self.__current_seconds = self.__total_time % 3600 % 24 % 365
def show_time(self):
print(f"Current hours {self.__current_hours}:{self.__current_minute}:{self.__current_seconds}")
def get_hours(self):
return self.__current_hours
def get_minute(self):
return self.__current_minute
def get_second(self):
return self.__current_seconds
a = time_show().get_second()
b = time_show().get_hours()
c = time_show().get_minute()
time_show().show_time()
def Draw_line(second, minute, hour):
turtle.speed(30)
axis_x = 0
axis_y = 0
turtle.penup()
turtle.goto(axis_x, axis_y - 150)
turtle.pendown()
turtle.circle(150)
turtle.penup()
turtle.goto(axis_x, axis_y)
turtle.dot(7, "blue")
turtle.penup()
turtle.goto(axis_x, axis_y)
turtle.right(270+second)
turtle.pendown()
turtle.color("red")
turtle.forward(100)
turtle.penup()
turtle.goto(axis_x, axis_y)
turtle.right(0)
turtle.penup()
turtle.pensize(5)
turtle.goto(axis_x, axis_y)
turtle.right(270+minute)
turtle.pendown()
turtle.color('blue')
turtle.forward(80)
turtle.penup()
turtle.goto(axis_x, axis_y)
turtle.right(0)
turtle.penup()
turtle.pensize(5)
turtle.goto(axis_x, axis_y)
turtle.right(270+hour)
turtle.pendown()
turtle.color('black')
turtle.forward(80)
turtle.penup()
turtle.goto(axis_x, axis_y)
turtle.right(0)
Draw_line(a, b, c)
turtle.hideturtle()
turtle.done()
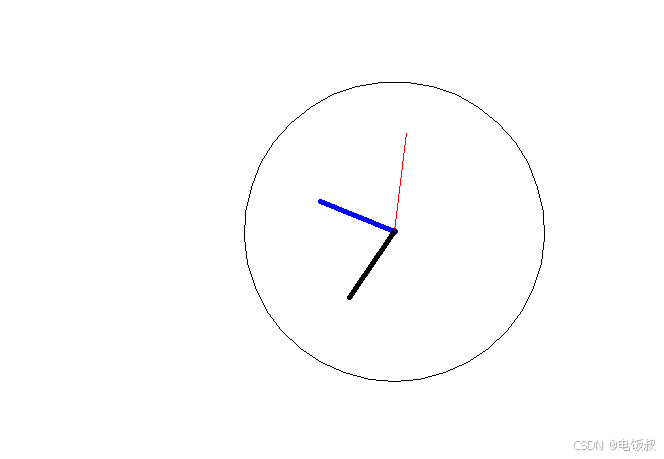
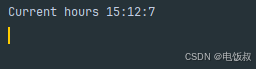